Getting proportionally-resized dimensions of an image
•
1 min read
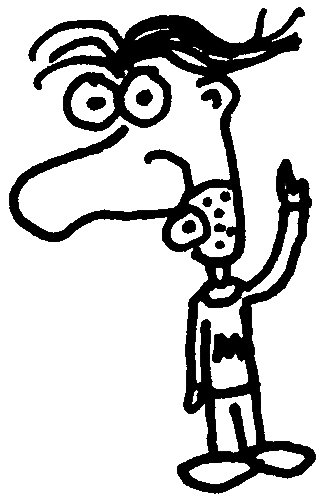
Heads up! This post was written in 2007, so it may contain information that is no longer accurate. I keep posts like this around for historical purposes and to prevent link rot, so please keep this in mind as you're reading.
— Cory
This is extremely useful when you need to scale down an image within a certain pair of dimensions.
get_resized_dimensions() #
Parameters #
$width
- The width of the image to be resized$height
- The height of the image to be resized$max_width
- The maximum allowed width of the resized image$max_height
- The maximum allowed height of the resized image
Return values #
The function outputs an array containing two elements: width
and height
$new_dimensions = get_resized_dimensions(640, 480, 200, 200)
echo $new_dimensions['width'] . ', ' . $new_dimensions['height'];
The output for the above code will be: 200, 150
Code #
function get_resized_dimensions($width, $height, $max_width, $max_height) {
// Check for bad values
if ($width <= 0 || $height <= 0) return false;
// Determine aspect ratio
$aspect_ratio = $height / $width;
// First check width
if ($width > $max_width) {
$new_width = $max_width;
$new_height = $new_width * $aspect_ratio;
} else {
$new_width = $width;
$new_height = $height;
}
// Now check height
if ($new_height > $max_height) {
$new_height = $max_height;
$new_width = $new_height / $aspect_ratio;
}
return array('width' => $new_width, 'height' => $new_height);
}